Struts 2 Annotation Example
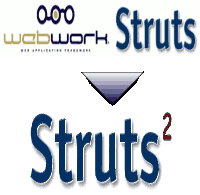
Struts 2 Annotation Example explains step by step example of configuring Struts 2 annotation with eclipse
Apache Struts 2, is a popular Java Model-View-Contraller (MVC) framework, which is developed by merging WebWork and Struts 1.x web frameworks.
Struts 2 have lot off difference with struts 1, struts 2 have implemented with an idea of convention over configuration, it also have many annotations in order to increase the developer productivity.
Features like interceptors, OGNL expression & value stack model are some of the benefits, you will get when you are developing with struts 2
Here we are using annotations inside Action class, so that we can remove struts.xml configuration file
Required Libraries
Following jar must be in classpath
- asm-3.3.jar
- asm-commons-3.3.jar
- commons-fileupload-1.3.jar
- commons-io-2.0.1.jar
- commons-lang3-3.1.jar
- commons-logging-1.1.3.jar
- freemarker-2.3.19.jar
- javassist-3.11.0.GA.jar
- ognl-3.0.6.jar
- struts2-convention-plugin-2.3.15.1.jar
- struts2-core-2.3.15.1.jar
- xwork-core-2.3.15.1.jar
You can see the project structure below
index.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%> <%@taglib uri="/struts-tags" prefix="s"%> <html> <body> <s:form action="HelloUser"> <s:textfield name="Name" label="Name" /> <s:submit /> </s:form> </body> </html>
success.jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%> <%@taglib uri="/struts-tags" prefix="s"%> <html> <body> <h1> <s:property value="message" /> </h1> </body> </html>
HelloUser.java
Here is the action class, logic is implemented here. You can see below Action tag
@Action(value = "HelloUser", results = { @Result(name = "result", location = "/success.jsp") })
Here @Action tag is used for mapping the request (ie; result) with correponding response (ie; success.jsp).
import org.apache.struts2.convention.annotation.Action;
import org.apache.struts2.convention.annotation.Result;
import org.apache.struts2.convention.annotation.ResultPath;
import com.opensymphony.xwork2.ActionSupport;
@ResultPath(value = "/")
public class HelloUser extends ActionSupport {
private static final long serialVersionUID = 1L;
private String message;
private String name;
@Action(value = "HelloUser", results = { @Result(name = "result", location = "/success.jsp") })
public String execute() {
setMessage("Hello " + getName());
return "result";
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <session-config> <session-timeout>30</session-timeout> </session-config> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
Run