Download DOC File Using CXF REST / JAX-RS
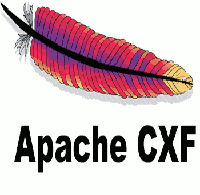
Download Doc File Using CXF REST / JAX-RS explains about downloading a Microsoft Word Document using CXF REST / JAX-RS API
@Produces("application/msword")
On the above annotation, we are mapping the response as application/msword mime type, you can change mime type according to different file formats
response.header("Content-Disposition", "attachment; filename=test.doc");
We are also setting header as "Content-Disposition" in order to open file as an attachment
This tutorial is for downloading Doc file, but you can see different mime types(formats) below
Download PDF File Using CXF REST / JAX-RS Download DOC File Using CXF REST / JAX-RS Download Excel File Using CXF REST / JAX-RS Download Image File Using CXF REST / JAX-RS Download Text File Using CXF REST / JAX-RS
Download Doc File Using CXF REST / JAX-RS
I am going to re-use CXF REST File Upload
We are making changes on UploadServiceImpl class, just adding new method downloadFile() in order to download Doc file
package com.student;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import javax.activation.DataHandler;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.ResponseBuilder;
import org.apache.cxf.jaxrs.ext.multipart.Attachment;
public class UploadServiceImpl {
private static final String FILE_PATH = "c:\\downloads\\test.doc";
@GET
@Path("/downloadFile")
@Produces("application/msword")
public Response downloadFile() {
File file = new File(FILE_PATH);
ResponseBuilder response = Response.ok((Object) file);
response.header("Content-Disposition", "attachment; filename=test.doc");
return response.build();
}
@POST
@Path("/uploadFile")
@Consumes(MediaType.MULTIPART_FORM_DATA)
public Response uploadFile(List<Attachment> attachments, @Context HttpServletRequest request) {
for (Attachment attachment : attachments) {
DataHandler handler = attachment.getDataHandler();
try {
InputStream stream = handler.getInputStream();
MultivaluedMap<String, String> map = attachment.getHeaders();
System.out.println("fileName Here" + getFileName(map));
OutputStream out = new FileOutputStream(new File("C:/uploads/" + getFileName(map)));
int read = 0;
byte[] bytes = new byte[1024];
while ((read = stream.read(bytes)) != -1) {
out.write(bytes, 0, read);
}
stream.close();
out.flush();
out.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return Response.ok("file uploaded").build();
}
private String getFileName(MultivaluedMap<String, String> header) {
String[] contentDisposition = header.getFirst("Content-Disposition").split(";");
for (String filename : contentDisposition) {
if ((filename.trim().startsWith("filename"))) {
String[] name = filename.split("=");
String exactFileName = name[1].trim().replaceAll("\"", "");
return exactFileName;
}
}
return "unknown";
}
}
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import javax.activation.DataHandler;
import javax.servlet.http.HttpServletRequest;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.Context;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.ResponseBuilder;
import org.apache.cxf.jaxrs.ext.multipart.Attachment;
public class UploadServiceImpl {
private static final String FILE_PATH = "c:\\downloads\\test.doc";
@GET
@Path("/downloadFile")
@Produces("application/msword")
public Response downloadFile() {
File file = new File(FILE_PATH);
ResponseBuilder response = Response.ok((Object) file);
response.header("Content-Disposition", "attachment; filename=test.doc");
return response.build();
}
@POST
@Path("/uploadFile")
@Consumes(MediaType.MULTIPART_FORM_DATA)
public Response uploadFile(List<Attachment> attachments, @Context HttpServletRequest request) {
for (Attachment attachment : attachments) {
DataHandler handler = attachment.getDataHandler();
try {
InputStream stream = handler.getInputStream();
MultivaluedMap<String, String> map = attachment.getHeaders();
System.out.println("fileName Here" + getFileName(map));
OutputStream out = new FileOutputStream(new File("C:/uploads/" + getFileName(map)));
int read = 0;
byte[] bytes = new byte[1024];
while ((read = stream.read(bytes)) != -1) {
out.write(bytes, 0, read);
}
stream.close();
out.flush();
out.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return Response.ok("file uploaded").build();
}
private String getFileName(MultivaluedMap<String, String> header) {
String[] contentDisposition = header.getFirst("Content-Disposition").split(";");
for (String filename : contentDisposition) {
if ((filename.trim().startsWith("filename"))) {
String[] name = filename.split("=");
String exactFileName = name[1].trim().replaceAll("\"", "");
return exactFileName;
}
}
return "unknown";
}
}
Run Client
You can test this service in two different ways
1) Using Java Program
package com.client;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
public class DownloadFileClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestUpload/services/rest/downloadFile");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("GET");
conn.setRequestProperty("Content-Type", "application/json");
if (conn.getResponseCode() == 200) {
InputStream inputStream = conn.getInputStream();
OutputStream output = new FileOutputStream("C:/downloads/copyOfTest.doc");
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
output.close();
} else {
Scanner scanner = new Scanner(conn.getErrorStream());
System.out.println(scanner.next());
scanner.close();
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
public class DownloadFileClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestUpload/services/rest/downloadFile");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("GET");
conn.setRequestProperty("Content-Type", "application/json");
if (conn.getResponseCode() == 200) {
InputStream inputStream = conn.getInputStream();
OutputStream output = new FileOutputStream("C:/downloads/copyOfTest.doc");
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
output.close();
} else {
Scanner scanner = new Scanner(conn.getErrorStream());
System.out.println(scanner.next());
scanner.close();
}
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output

2) Using Browser