CXF Restful Client
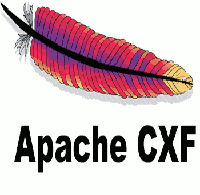
CXF Restful Client Example explains step by step details of How To Create a CXF REST Client from a deployed Apache CXF Restful service
For Creating Apache CXF Restful Client, We are using java.net.HttpURLConnection.
By using HttpURLConnection, we are invoking the correct exposed methods / operations of restful service.
You can see the below example, which is demonstrating a CXF REST Client Example
Create CXF Restful Client
Here we showing an example of a CXF REST client, which invoking a POST method of a CXF Restful Service
package com.client;
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// CXF REST Client Invoking POST Method
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// CXF REST Client Invoking POST Method
public class PostStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/changeName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "application/json");
String input = "{\"Student\":{\"name\":\"Tom\"}}";
OutputStream os = conn.getOutputStream();
os.write(input.getBytes());
os.flush();
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
Response From Server{"Student":{"name":"HELLO Tom"}}
Here we showing an example of a CXF REST client, which invoking a GET method of a CXF Restful Service
package com.client;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// CXF JAX-RS client Invoking GET Method
public class GetStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/getName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("GET");
conn.setRequestProperty("Content-Type", "application/json");
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Scanner;
// CXF JAX-RS client Invoking GET Method
public class GetStudentClient {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/CXFRestfulTutorial/rest/getName");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setRequestMethod("GET");
conn.setRequestProperty("Content-Type", "application/json");
Scanner scanner;
String response;
if (conn.getResponseCode() != 200) {
scanner = new Scanner(conn.getErrorStream());
response = "Error From Server \n\n";
} else {
scanner = new Scanner(conn.getInputStream());
response = "Response From Server \n\n";
}
scanner.useDelimiter("\\Z");
System.out.println(response + scanner.next());
scanner.close();
conn.disconnect();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output
Response From Server{"Student":{"name":"Rockey"}}
2 Responses to "CXF Restful Client"