Getting Mime type in Java
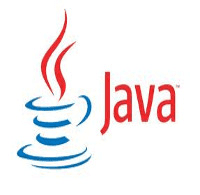
In this tutorial, I am showing how to get mime type of a file in java using Java 7 Files and URLConnection class.
You can select right one from the below listed, which suits your
needs.
Using Java 7 Files.probeContentType
// using java 7 probeContentType String mimeType = Files.probeContentType(path); System.out.println(mimeType);
Using With URLConnection getContentType
// getContentType URLConnection connection = file.toURL().openConnection(); System.out.println(connection.getContentType());
Using URLConnection guessContentTypeFromName
// guessContentTypeFromName System.out.println(URLConnection.guessContentTypeFromName(file.getName()));
Using URLConnection getFileNameMap
// getFileNameMap System.out.println(URLConnection.getFileNameMap().getContentTypeFor(file.getName()));
You can find the complete example of finding mimes type of a file below with all the above described ways.
package com.javatips; import java.io.File; import java.io.IOException; import java.net.URLConnection; import java.nio.file.Files; import java.nio.file.Path; public class MimeExample { public static void main(String[] args) throws IOException { File file = new File("java.png"); Path path = file.toPath(); // using java 7 probeContentType String mimeType = Files.probeContentType(path); System.out.println(mimeType); // getContentType URLConnection connection = file.toURL().openConnection(); System.out.println(connection.getContentType()); // guessContentTypeFromName System.out.println(URLConnection.guessContentTypeFromName(file.getName())); // getFileNameMap System.out.println(URLConnection.getFileNameMap().getContentTypeFor(file.getName())); } }
Output
image/png image/png image/png image/png