CXF With JBoss Tutorial
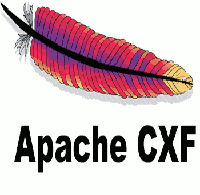
CXF With JBoss Tutorial explains about the integration of CXF Framework with Jboss server.
Apache CXF is a free and open source project, and a fully featured Webservice framework.It helps you building webservices using different front-end API's, like as JAX-RS and JAX-WS.
Reference → https://cxf.apache.org/
Required Libraries
You need to download
Following jar must be in classpath
- aopalliance-1.0.jar
- asm-5.0.4.jar
- cxf-core-3.1.4.jar
- cxf-rt-bindings-soap-3.1.4.jar
- cxf-rt-databinding-jaxb-3.1.4.jar
- cxf-rt-frontend-jaxws-3.1.4.jar
- cxf-rt-frontend-simple-3.1.4.jar
- cxf-rt-transports-http-3.1.4.jar
- cxf-rt-ws-policy-3.1.4
- cxf-rt-wsdl-3.1.4.jar
- jaxb-core-2.2.11.jar
- jaxb-impl-2.2.11.jar
- jcl-over-slf4j-1.7.12.jar
- neethi-3.0.3.jar
- slf4j-api-1.7.12.jar
- slf4j-jdk14-1.7.12.jar
- spring-aop-4.1.7.RELEASE.jar
- spring-beans-4.1.7.RELEASE.jar
- spring-context-4.1.7.RELEASE.jar
- spring-core-4.1.7.RELEASE.jar
- spring-expression-4.1.7.RELEASE.jar
- spring-web-4.1.7.RELEASE.jar
- stax2-api-3.1.4.jar
- woodstox-core-asl-4.4.1.jar
- wsdl4j-1.6.3.jar
- xml-resolver-1.2.jar
- xmlschema-core-2.2.1.jar
CXF JBoss Tutorial
I am creating a sample web service project that pass Student object and return with some changes on that object. The service is using simple POJO (Plain Old Java Object) bean.
Firstly create a Dynamic Web Project (File->New->Dynamic Web Project) named "CXFTutorial" according to following screenshot
Create a Student Object
public class Student {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Create a Service Interface
This service interface will defines which methods of web service, to be invoked by the client
import javax.jws.WebService;
@WebService
public interface ChangeStudentDetails {
Student changeName(Student student);
}
Implement the Service Interface
Here we implement the service interface created on the previous step
import javax.jws.WebService;
@WebService(endpointInterface = "com.student.ChangeStudentDetails")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
public Student changeName(Student student) {
student.setName("Hello "+student.getName());
return student;
}
}
Create a cxf.xml
CXF is using Spring internally, Finding classes by spring we need to add service implementation class on "jaxws:endpoint" tag
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xmlns:soap="http://cxf.apache.org/bindings/soap" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/bindings/soap http://cxf.apache.org/schemas/configuration/soap.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"> <jaxws:endpoint id="changeStudent" implementor="com.student.ChangeStudentDetailsImpl" address="/ChangeStudent" /> </beans>
Change web.xml
Change the web.xml file to find CXF servlet and cxf.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <context-param> <param-name>contextConfigLocation</param-name> <param-value>WEB-INF/cxf.xml</param-value> </context-param> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <servlet> <servlet-name>CXFServlet</servlet-name> <servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>CXFServlet</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping> </web-app>
Publishing CXF Web Service
Deployed CXF Web Service