CXF java.net.SocketTimeoutException: Read timed out
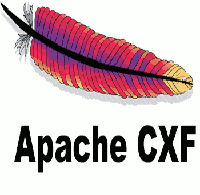
This exception happens, when invoking the client and it takes 50-60 seconds after that we get java.net.SocketTimeoutException: Read timed out, following are the stacktrace
Caused by: org.apache.cxf.interceptor.Fault: Could not send Message.
at org.apache.cxf.interceptor.MessageSenderInterceptor$MessageSenderEndingInterceptor.handleMessage
(MessageSenderInterceptor.java:64)
at org.apache.cxf.phase.PhaseInterceptorChain.doIntercept(PhaseInterceptorChain.java:220)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:276)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:222)
at org.apache.cxf.frontend.ClientProxy.invokeSync(ClientProxy.java:73)
at org.apache.cxf.jaxws.JaxWsClientProxy.invoke(JaxWsClientProxy.java:171)
... 26 more
Caused by: java.net.SocketTimeoutException: Read timed out
at java.net.SocketInputStream.socketRead0(Native Method)
at java.net.SocketInputStream.read(SocketInputStream.java:129)
at java.io.BufferedInputStream.fill(BufferedInputStream.java:218)
at java.io.BufferedInputStream.read1(BufferedInputStream.java:258)
at java.io.BufferedInputStream.read(BufferedInputStream.java:317)
This article explains about how to fix this exception in CXF way
Required Libraries
You need to download following libraries in order to Generate CXF Client
Following jar must be in classpath
- cxf-core-3.3.6.jar
- cxf-rt-bindings-soap-3.3.6.jar
- cxf-rt-databinding-jaxb-3.3.6.jar
- cxf-rt-features-logging-3.3.6.jar
- cxf-rt-frontend-jaxws-3.3.6.jar
- cxf-rt-frontend-simple-3.3.6.jar
- cxf-rt-transports-http-3.3.6.jar
- cxf-rt-wsdl-3.3.6.jar
- httpasyncclient-4.1.4.jar
- httpclient-4.5.12.jar
- httpcore-4.4.13.jar
- httpcore-nio-4.4.13.jar
- neethi-3.1.1.jar
- slf4j-api-1.7.29.jar
- slf4j-jdk14-1.7.29.jar
- spring-aop-5.1.14.RELEASE.jar
- spring-beans-5.1.14.RELEASE.jar
- spring-context-5.1.14.RELEASE.jar
- spring-core-5.1.14.RELEASE.jar
- spring-expression-5.1.14.RELEASE.jar
- spring-jcl-5.1.14.RELEASE.jar
- spring-web-5.1.14.RELEASE.jar
- stax2-api-3.1.4.jar
- woodstox-core-5.0.3.jar
- wsdl4j-1.6.3.jar
- xmlschema-core-2.2.5.jar
Fix java.net.SocketTimeoutException: Read timed out exception
You can use HTTPConduit and HTTPClientPolicy and need to set ConnectionTimeout and ReceiveTimeout when invoking the service, please see the below code
Run Client
import org.apache.cxf.frontend.ClientProxy;
import org.apache.cxf.interceptor.LoggingInInterceptor;
import org.apache.cxf.interceptor.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import org.apache.cxf.transport.http.HTTPConduit;
import org.apache.cxf.transports.http.configuration.HTTPClientPolicy;
import com.student.ChangeStudentDetails;
import com.student.Student;
// How to handle java.net.SocketTimeoutException: Read timed out exception
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
long timeout = 10000L;
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8080/CXFTutorial/ChangeStudent");
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
ChangeStudentDetails ChangeStudentService = (ChangeStudentDetails) factory.create();
Client client = ClientProxy.getClient(ChangeStudentService);
if (client != null) {
HTTPConduit conduit = (HTTPConduit) client.getConduit();
HTTPClientPolicy policy = new HTTPClientPolicy();
policy.setConnectionTimeout(timeout);
policy.setReceiveTimeout(timeout);
conduit.setClient(policy);
}
Student student = new Student();
student.setName(Rockey);
Student changeName = ChangeStudentService.changeName(student);
System.out.println(Server said: + changeName.getName());
System.exit(0);
}
}
Output
Server said: Hello Rockey