CXF Asynchronous Client
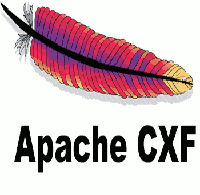
CXF Asynchronous Client tells about creating a SOAP/WSDL asynchronous client using Apache CXF WebService Framework
There are two options available for receiving data through asynchronous calls from client with CXF.
1) Polling: the client application periodically polls a javax.xml.ws.Response instance to check if the response is available
2) Callback: the client application implements the javax.xml.ws.AsyncHandler interface to accept notification of the response availability
Reference -> http://cxf.apache.org/docs/developing-a-consumer.html
Below we are showing examples for both approaches
Required Libraries
You need to download following libraries in order to Generate Asynchronous CXF Client
- JDK 6
- Eclipse 3.7
- CXF-2.7.3
- Tomcat 7 (Deploying Web Service)
Following jar must be in classpath
- commons-logging-1.1.1.jar
- cxf-2.7.3.jar
- httpasyncclient-4.0-beta3.jar
- httpclient-4.2.1.jar
- httpcore-4.2.2.jar
- httpcore-nio-4.2.2.jar
- neethi-3.0.2.jar
- spring-aop-3.0.7.RELEASE.jar
- spring-asm-3.0.7.RELEASE.jar
- spring-beans-3.0.7.RELEASE.jar
- spring-context-3.0.7.RELEASE.jar
- spring-core-3.0.7.RELEASE.jar
- spring-expression-3.0.7.RELEASE.jar
- spring-web-3.0.7.RELEASE.jar
- wsdl4j-1.6.2.jar
- xmlschema-core-2.0.3.jar
1. CXF Asynchronous Client Using Polling
The client application periodically checks (poll) whether response is available from the server.
import javax.xml.ws.Response;
import org.apache.cxf.interceptor.LoggingInInterceptor;
import org.apache.cxf.interceptor.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import com.student.ChangeStudentDetails;
import com.student.Student;
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8081/CXFTutorial/ChangeStudent?wsdl");
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
ChangeStudentDetails client = (ChangeStudentDetails) factory.create();
// polling method
System.out.println("Invoking changeStudentAsync using polling...");
Response<Student> changeStudentResp = client.changeStudentAsync(System.getProperty("user.name"));
while (true) {
if (changeStudentResp.isDone()) {
Student reply = changeStudentResp.get();
System.out.println("Server responded through polling with: "
+ reply.getName());
break;
} else {
Thread.sleep(1000);
System.out.println("Polling %%%%%%%%%%%%%%%");
}
}
System.exit(0);
}
}
Output
Invoking changeStudentAsync using polling... Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Polling %%%%%%%%%%%%%%% Server responded through polling with: How are you Rockey
2. CXF Asynchronous Client Using Callback
The client application get responses from the server when response is ready, there is no polling happens here.
import java.util.concurrent.Future;
import org.apache.cxf.interceptor.LoggingInInterceptor;
import org.apache.cxf.interceptor.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import com.student.ChangeStudentDetails;
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8081/CXFTutorial/ChangeStudent?wsdl");
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
ChangeStudentDetails client = (ChangeStudentDetails) factory.create();
// callback method
TestAsyncHandler testAsyncHandler = new TestAsyncHandler();
System.out.println("Invoking changeStudentAsync using callback object...");
Future<?> response = client.changeStudentAsync(
System.getProperty("user.name"), testAsyncHandler);
while (!response.isDone()) {
Thread.sleep(100);
}
String resp = testAsyncHandler.getResponse();
System.out.println("Server responded through callback with: " + resp);
System.exit(0);
}
}
AsyncHandler Callback Implementation
import javax.xml.ws.AsyncHandler;
import javax.xml.ws.Response;
import com.student.Student;
public class TestAsyncHandler implements AsyncHandler<Student> {
private Student reply;
public void handleResponse(Response<Student> response) {
try {
System.err.println("handleResponse called");
reply = response.get();
} catch (Exception ex) {
ex.printStackTrace();
}
}
public String getResponse() {
return reply.getName();
}
}
Output
Invoking changeStudentAsync using callback object... Server responded through callback with: How are you Rockey