Convert Java String to InputStream
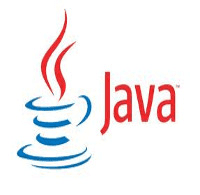
In this tutorial, I am showing how to convert Java String to InputStream using plain Java, Java 8 and Apache Commons IO library.
An InputStream
is connected to some data streams
like a file, memory
bufferes, network
connection, pipe etc. It is
used for reading the data from above sources.
Convert String to InputStream Using JDK
import java.io.ByteArrayInputStream; import java.io.IOExce⁞ption; import java.io.InputStream; import java.nio.charset.StandardCharsets; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(hello.getBytes(StandardCharsets.UTF_8)); } }
Convert String to InputStream Using Java 8
import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.nio.charset.StandardCharsets; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = new ByteArrayInputStream(StandardCharsets.UTF_8.encode(hello).array()); } }
Convert String to InputStream Using Apache Commons IOUtils
import java.io.IOException; import java.io.InputStream; import org.apache.commons.io.IOUtils; public class Test { public static void main(String[] args) throws IOException { String hello = "hello world"; InputStream is = IOUtils.toInputStream(hello); } }