Apache CXF Logging
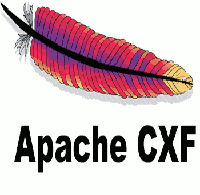
Apache CXF Logging explains about configure / enable logging of request and response using CXF framework
For every application logging is vital because it is a must for debugging web services, CXF framework have in-built feature for enabling logging of request / response
You can Enable / Configure CXF Logging using two ways
1) Server Side Logging 2) Client Side Logging
If you are interested to add logs in separate file using log4j, you can find below article
CXF Server Side Logging
Here I am going to re-use CXF Web Service Tutorial
You can enable Server Side Logging using two ways
1) Using Interceptor 2) Using cxf:features
For enabling server side logging using Interceptor you need to add logInInterceptor & logOutInterceptor
You need to import xmlns:cxf namespace in order to use <cxf:bus>
please check the following changed cxf.xml
cxf.xml
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:cxf="http://cxf.apache.org/core" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xsi:schemaLocation="http://cxf.apache.org/core http://cxf.apache.org/schemas/core.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"> <import resource="classpath:META-INF/cxf/cxf.xml" /> <bean class="org.apache.cxf.ext.logging.LoggingInInterceptor" id="logInInterceptor" /> <bean class="org.apache.cxf.ext.logging.LoggingOutInterceptor" id="logOutInterceptor" /> <cxf:bus> <cxf:inInterceptors> <ref bean="logInInterceptor" /> </cxf:inInterceptors> <cxf:outInterceptors> <ref bean="logOutInterceptor" /> </cxf:outInterceptors> </cxf:bus> <jaxws:endpoint id="changeStudent" implementor="com.student.ChangeStudentDetailsImpl" address="/ChangeStudent" /> </beans>
Or you can use the following cxf.xml configuration using <cxf:features>
cxf.xml
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:cxf="http://cxf.apache.org/core" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xsi:schemaLocation="http://cxf.apache.org/core http://cxf.apache.org/schemas/core.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"> <import resource="classpath:META-INF/cxf/cxf.xml" /> <cxf:bus> <cxf:features> <cxf:logging /> </cxf:features> </cxf:bus> <jaxws:endpoint id="changeStudent" implementor="com.student.ChangeStudentDetailsImpl" address="/ChangeStudent" /> </beans>
Now just run this below client
import org.apache.cxf.ext.logging.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import com.student.ChangeStudentDetails;
import com.student.Student;
// Invoke CXF Server Side Logging
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8080/CXFTutorial/ChangeStudent?wsdl");
ChangeStudentDetails client = (ChangeStudentDetails) factory.create();
Student student = new Student();
student.setName("Rockey");
Student changeName = client.changeName(student);
System.out.println("Server said: " + changeName.getName());
System.exit(0);
}
}
Output
Here you can see the Tomcat server started and showing the request/response payloads
CXF Client Side Logging
Just run this below client you can see that we are adding the built in interceptors on client side
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
import org.apache.cxf.ext.logging.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import com.student.ChangeStudentDetails;
import com.student.Student;
// Invoke CXF Client Side Logging
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8080/CXFTutorial/ChangeStudent?wsdl");
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
ChangeStudentDetails client = (ChangeStudentDetails) factory.create();
Student student = new Student();
student.setName("Rockey");
Student changeName = client.changeName(student);
System.out.println("Server said: " + changeName.getName());
System.exit(0);
}
}
Output
Here you can see that Eclipse console is showing request/response payloads