CXF Web Service Tutorial
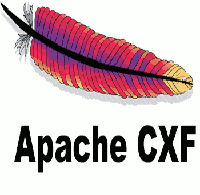
CXF Web Service Tutorial explains about step by step details of Creating / Developing Web service using Apache CXF, Spring & Eclipse and deployed in Tomcat
Apache CXF is a free and open source project, and a fully featured Webservice framework.
It helps you building webservices using different front-end API's, like as JAX-RS and JAX-WS.
Services will talk different protocols such as SOAP, RESTful HTTP, CORBA & XML/HTTP and work with different transports like JMS, HTTP or JBI.
Apache CXF Project was created by the merger of the Celtix and XFire projects. These two projects were merged by folks working together at the Apache Software Foundation.
Required Libraries
You need to download
Following jar must be in classpath
- cxf-core-3.3.6.jar
- cxf-rt-bindings-soap-3.3.6.jar
- cxf-rt-databinding-jaxb-3.3.6.jar
- cxf-rt-features-logging-3.3.6.jar
- cxf-rt-frontend-jaxws-3.3.6.jar
- cxf-rt-frontend-simple-3.3.6.jar
- cxf-rt-transports-http-3.3.6.jar
- cxf-rt-wsdl-3.3.6.jar
- httpasyncclient-4.1.4.jar
- httpclient-4.5.12.jar
- httpcore-4.4.13.jar
- httpcore-nio-4.4.13.jar
- neethi-3.1.1.jar
- slf4j-api-1.7.29.jar
- slf4j-jdk14-1.7.29.jar
- spring-aop-5.1.14.RELEASE.jar
- spring-beans-5.1.14.RELEASE.jar
- spring-context-5.1.14.RELEASE.jar
- spring-core-5.1.14.RELEASE.jar
- spring-expression-5.1.14.RELEASE.jar
- spring-jcl-5.1.14.RELEASE.jar
- spring-web-5.1.14.RELEASE.jar
- stax2-api-3.1.4.jar
- woodstox-core-5.0.3.jar
- wsdl4j-1.6.3.jar
- xmlschema-core-2.2.5.jar
CXF Tutorial
I am creating a sample web service project that pass Student object and return with some changes on that object. The service is using simple POJO (Plain Old Java Object) bean.
Firstly create a Dynamic Web Project (File->New->Dynamic Web Project) named "CXFTutorial" according to following screenshot
Create a Student Object
public class Student {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Create a Service Interface
This service interface will defines which methods of web service, to be invoked by the client
import javax.jws.WebService;
@WebService
public interface ChangeStudentDetails {
Student changeName(Student student);
}
Implement the Service Interface
Here we implement the service interface created on the previous step
import javax.jws.WebService;
@WebService(endpointInterface = "com.student.ChangeStudentDetails")
public class ChangeStudentDetailsImpl implements ChangeStudentDetails {
public Student changeName(Student student) {
student.setName("Hello "+student.getName());
return student;
}
}
Create a cxf.xml
CXF is using Spring internally, Finding classes by spring we need to add service implementation class on "jaxws:endpoint" tag
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxws="http://cxf.apache.org/jaxws" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://cxf.apache.org/jaxws http://cxf.apache.org/schemas/jaxws.xsd"> <jaxws:endpoint id="changeStudent" implementor="com.student.ChangeStudentDetailsImpl" address="/ChangeStudent" /> </beans>
Change web.xml
Change the web.xml file to find CXF servlet and cxf.xml
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <context-param> <param-name>contextConfigLocation</param-name> <param-value>WEB-INF/cxf.xml</param-value> </context-param> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <servlet> <servlet-name>CXFServlet</servlet-name> <servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>CXFServlet</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping> </web-app>
Publishing CXF Web Service
Deployed CXF Web Service