Create CXF Client
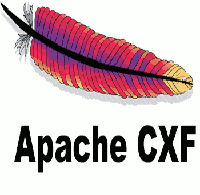
Create CXF Client Example explains step by step details of how to create a CXF web service client / wsdl soap client using Apache CXF WebService Framework
For Creating Apache CXF Client, We are using org.apache.cxf.jaxws.JaxWsProxyFactoryBean. The factory option is particular to CXF and it is non-standard. This will give you a great control over the endpoint.
The Other options like Wsimport tool available with JDK are standard but that will give you less control. For example you can add a logging interceptor in order to show the logs when accessing your service using org.apache.cxf.jaxws.JaxWsProxyFactoryBean.
For publishing a web service, you can follow this tutorial CXF Web Service Tutorial. This client is based on mentioned Tutorial
Required Libraries
You need to download following libraries in order to Generate CXF Client
- OpenJDK 8 / Corretto
- Eclipse 4.15
- CXF-3.3.6
- Tomcat 9 (Deploying Web Service)
Following jar must be in classpath
- cxf-core-3.3.6.jar
- cxf-rt-bindings-soap-3.3.6.jar
- cxf-rt-databinding-jaxb-3.3.6.jar
- cxf-rt-features-logging-3.3.6.jar
- cxf-rt-frontend-jaxws-3.3.6.jar
- cxf-rt-frontend-simple-3.3.6.jar
- cxf-rt-transports-http-3.3.6.jar
- cxf-rt-wsdl-3.3.6.jar
- httpasyncclient-4.1.4.jar
- httpclient-4.5.12.jar
- httpcore-4.4.13.jar
- httpcore-nio-4.4.13.jar
- neethi-3.1.1.jar
- slf4j-api-1.7.29.jar
- slf4j-jdk14-1.7.29.jar
- spring-aop-5.1.14.RELEASE.jar
- spring-beans-5.1.14.RELEASE.jar
- spring-context-5.1.14.RELEASE.jar
- spring-core-5.1.14.RELEASE.jar
- spring-expression-5.1.14.RELEASE.jar
- spring-jcl-5.1.14.RELEASE.jar
- spring-web-5.1.14.RELEASE.jar
- stax2-api-3.1.4.jar
- woodstox-core-5.0.3.jar
- wsdl4j-1.6.3.jar
- xmlschema-core-2.2.5.jar
Create CXF Client
import org.apache.cxf.ext.logging.LoggingOutInterceptor;
import org.apache.cxf.jaxws.JaxWsProxyFactoryBean;
import com.student.ChangeStudentDetails;
import com.student.Student;
// CXF JAX-WS Client / Consuming Web Services With CXF
public final class StudentClient {
public static void main(String args[]) throws Exception {
JaxWsProxyFactoryBean factory = new JaxWsProxyFactoryBean();
factory.setServiceClass(ChangeStudentDetails.class);
factory.setAddress("http://localhost:8080/CXFTutorial/ChangeStudent?wsdl");
factory.getInInterceptors().add(new LoggingInInterceptor());
factory.getOutInterceptors().add(new LoggingOutInterceptor());
ChangeStudentDetails client = (ChangeStudentDetails) factory.create();
Student student = new Student();
student.setName("Rockey");
Student changeName = client.changeName(student);
System.out.println("Server said: " + changeName.getName());
System.exit(0);
}
}
Output
Server said: Hello Rockey