Call Jasper Report / Ireport From Java Application

Call Jasper Report / Ireport From Java Application Example describes about How to call Jasper Report and Ireport from a java application.
Jasper-Reports is a free reporting engine that could be written to screen, or to a printer or as HTML, PDF, RTF, Microsoft Excel, XML files, CSV format and ODT files.
Jasper-Report is using in Java related applications, including Web and Enterprise applications, to develop dynamic reports. It reads its instructions from jasper file (compiled) or XML dynamically
We can generate reports using two ways,
1) From "JRXML" (Source) file
2) From "Jasper" (Compiled) file
Required Libraries
You need to download
- JDK 6
- iReport-4.1.1 for designing the report
Following jar must be in classpath (Available from ireport installation directory)
- commons-beanutils-1.8.2.jar
- commons-collections-3.2.1.jar
- commons-digester-1.7.jar
- commons-logging-1.1.jar
- groovy-all-1.7.5.jar
- iText-2.1.7.jar
- jasperreports-4.1.1.jar
1) Create PDF Report From JRXML File
JRXML file is a JasperReports Document. JRXML is the XML file format of JasperReport, which can be coded manually, generated, or created using a tools like IReport, JasperAssistant etc
Execution of report from JRXML file will be very slow, as it need to compile before the execution
import java.util.HashMap;
import net.sf.jasperreports.engine.JREmptyDataSource;
import net.sf.jasperreports.engine.JRException;
import net.sf.jasperreports.engine.JasperExportManager;
import net.sf.jasperreports.engine.JasperFillManager;
import net.sf.jasperreports.engine.JasperPrint;
// Java Program To Call Jasper Report
public class PdfFromXmlFile {
public static void main(String[] args) throws JRException, IOException {
JasperReport jasperReport = JasperCompileManager.compileReport("report.jrxml");
JasperPrint jasperPrint = JasperFillManager.fillReport(jasperReport,new HashMap(), new JREmptyDataSource());
JasperExportManager.exportReportToPdfFile(jasperPrint, "sample.pdf");
}
}
Output
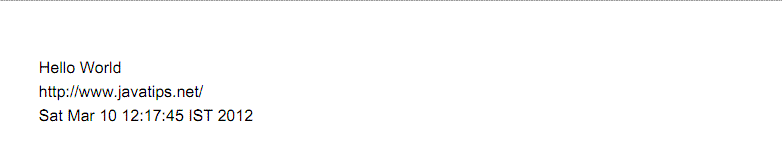
2) Create PDF Report From Jasper File
import java.util.HashMap;
import net.sf.jasperreports.engine.JREmptyDataSource;
import net.sf.jasperreports.engine.JRException;
import net.sf.jasperreports.engine.JasperExportManager;
import net.sf.jasperreports.engine.JasperFillManager;
import net.sf.jasperreports.engine.JasperPrint;
// How To Invoke Ireport From Java Application
public class PdfFromJasperFile {
public static void main(String[] args) throws JRException, IOException {
JasperPrint jasperPrint = JasperFillManager.fillReport("report.jasper", new HashMap<String, Object>(),
new JREmptyDataSource());
JasperExportManager.exportReportToPdfFile(jasperPrint, "sample.pdf");
}
}
Output
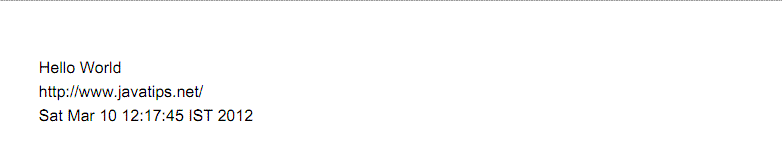